Getting Started
The Datablist REST API is exposed at https://data.datablist.com
. You must always connect to the API using HTTPS.
Our API has resource-oriented URLs, accepts JSON-encoded request bodies, returns JSON-encoded responses, uses standard HTTP response codes, and verbs.
#
AuthenticationEvery api endpoint requires an Authorization
header with an access token. We use self contained JWT access tokens sent using the Bearer format.
A valid API call looks like this:
Because our access token are self contained, they can be decoded to retrieve important information like user id, scope or expiration.
info
Our access tokens expire after 24h and must be refreshed.
#
Get Access Token from Personal API KeyPersonal API Keys let you generate access tokens. To create a Personal API Key, go to the API page in your Datablist account.
To get an access token, you must call the token
endpoint on https://account.datablist.com
.
If your Personal API Key is valid, the response will be:
Then use, the access_token
as Bearer
for your calls.
#
Requests and Responses formatThe only accepted content types are JSON and JSON-LD. Requests must use the Accept: application/json
or Accept: application/ld+json
(what format you want to receive) and Content-Type: application/json
or Content-Type: application/ld+json
(what content format are you sending in) headers.
Responses from Datablist are returned in json
with the Content-Type: application/json
or Content-Type: application/ld+json
header according to the Accept
header.
#
RequestsA request with a body looks like:
#
Responses#
HTTP Status CodesWe use HTTP Status Codes to inform on response status. Any code between 200 (included) and 300 (excluded) indicates the request has been successfully processed. Other codes indicate an error.
HTTP Status Code | Description |
---|---|
200 | Success. |
201 | Created object success. |
204 | Success with empty response. This is used for update (PUT, PATCH) and delete requests. |
400 | Bad Request |
401 | Unauthorized. Access Token is missing or invalid. |
404 | The resource could not be found. |
405 | Method Not Allowed for this endpoint. |
500 | Unexpected server error ๐ค. |
#
Error ResponsesOn error, a json response is returned with a HTTP status code and the following attributes:
Attribute | Description |
---|---|
http_status | Duplicate of the HTTP status code. |
code | A short string indicating the error reported. |
description | Human readable description of the error. |
#
Get, List, Create, Update, Delete - Single operation or bulkDatablist uses HTTP verbs for CRUD operations:
HTTP Verb | Description | Return |
---|---|---|
POST | Create an object | Return HTTP status 201 with a {"@id": "object_id"} response and a Location header pointing to the newly created resource |
GET | Retrieve object(s) | Return HTTP status 200. |
PATH | Partial Update | Return HTTP status 204 (empty response) |
PUT | Full Update | Return HTTP status 204 (empty response) |
DELETE | Delete object | Return HTTP status 204 (empty response) |
#
CreateThe createdAt
and @id
value can be set on a POST
request. If not provided, they will be auto generated.
If an object already exist with the same @id
, an error will be raised (http_status 400 and IntegrityError code).
Success response has a 201 status with the resource URI in the Location
http header.
#
UpdateupdatedAt
is updated on any PUT
or PATCH
request.
Success response returns a 204 status with an empty body.
#
BulkItem
endpoints accept bulk
operations with the {{endpoing}}/bulk
path. See documentation.
If you need to perform a high number of operations, we recommend to use both bulk request and parallelization.
Our current benchmarks recommend a maximum of 20 concurrency requests of 200 bulk operations.
Example: To create 10 000 items, you would perform 10000/200=50 requests with a maximum of 20 parallel requests.
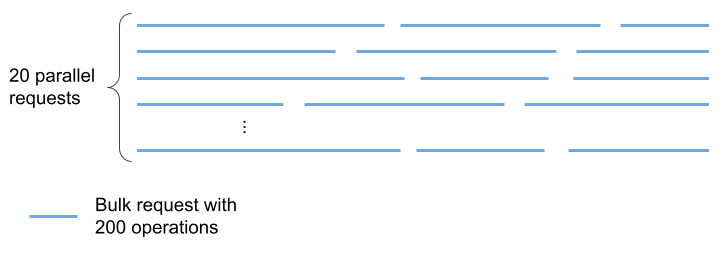
#
FilteringTo filter, add the filter
GET param with a list of filtering operators encoded using json.
Valid operators are:
- eq: Equals
- array_contains: Array Contains
- in: In
- not-in: Not In
- ge: Greater Than or Equal
Futur operators (not implemented):
- ne: Not Equals
- gt: Greater Than
- lt: Less Than
- l: Less Than or Equal
#
OrderingUse the order_by
GET param and the name of the field to order results. Use -
for descending.
Example:
or descending:
#
FAQ- Do you have API versioning? - You might notice we don't have versioning prefix on our API endpoints. We are guided with the Linked Data principles that requires that a resource object has one and only one URI. This is not compatible with versioning as part of the URI. The API is currently in Alpha and is likely to change in the coming months. Later, we will introduce versioning using headers.